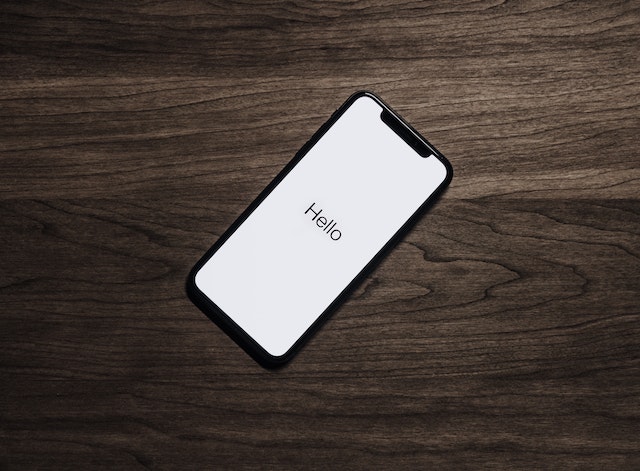
Coding | Tutorial Android
How to Make Splash Screen in Android Studio Using Kotlin
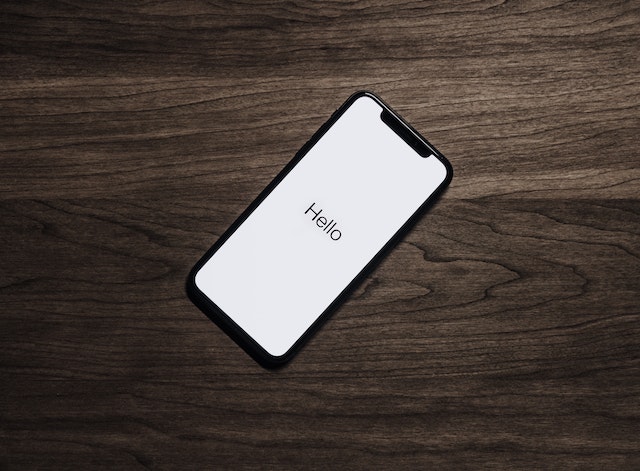
To create a splash screen in Android Studio using Kotlin, you can follow these steps:
- Create a new Android Studio project and select the “Empty Activity” template.
- In the “res” directory, create a new directory called “drawable” and add a splash screen image to this directory. This image should be the same size as the screen resolution of the device on which the app will be displayed.
- In the “styles.xml” file, which is located in the “res/values” directory, add a new style for the splash screen theme. This theme should have a transparent background and should reference the splash screen image as the background.
<style name="SplashTheme" parent="Theme.AppCompat.NoActionBar">
<item name="android:windowBackground">@drawable/splash_screen</item>
</style>
- In the “AndroidManifest.xml” file, set the splash screen theme as the app’s starting theme.
<activity android:name=".MainActivity"
android:theme="@style/SplashTheme">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
- In the “MainActivity.kt” file, set the app’s regular theme as the activity’s theme after a short delay. This will allow the splash screen to be displayed for a few seconds before the app’s main activity is displayed.
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
Handler().postDelayed({
// Set app theme after delay
setTheme(R.style.AppTheme)
}, SPLASH_SCREEN_DELAY)
}
}
- In the “res/values” directory, create a new resource file called “dimens.xml” and add a value for the splash screen delay.
<dimen name="splash_screen_delay">3000</dimen>
- In the “MainActivity.kt” file, define a constant for the splash screen delay and use the value from the “dimens.xml” file.
private const val SPLASH_SCREEN_DELAY = 3000L
- Run the app to see the splash screen.
No Comment